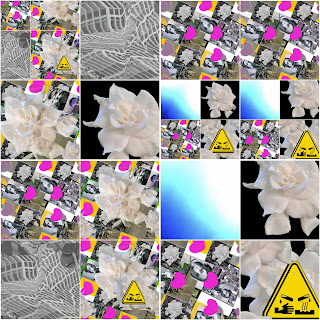 |
With Xavier Dallet aka chan-something star
|
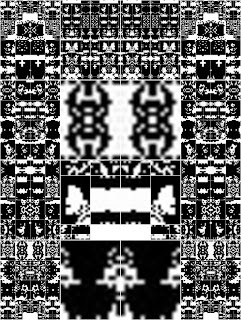 |
Whoppa whoppa whoppa
|
Ever wanted to take a series of images and make grids from them? Ever wanted to loop over the images and create ever more fractured but yet somehow consistent glitchy grids? Then this script is for you. Inspired by Xavier Dallet aka chan somethingstar and his Instagram group Baobab users https://www.instagram.com/baobab_users/
Requires imagemagick, bash and a basic knowledge of how to run shell scripts. Linux Script below. Name it and save with .sh extension. Modified version for windows 10+ using git bash, after the linux version - I've tested both as working.
#! /bin/bash
#make directory to hold output
h=$(pwd)
echo $h
#make folder to store originals
mkdir $h/originals
mkdir $h/frames
#make folder to hold output
mkdir rotator
#get formats to use
echo -n "Source image format ? : "
read f
echo -n "Image format to use ? : "
read t
echo -n "Discard cut in ? : "
read disin
echo -n "No to discard ? : "
read dis
#get grid dimensions
echo -n "Grid height ? : "
read gw
echo -n "Grid width ? : "
read gh
echo -n "Number of loops ? : "
read lo
echo -n "Use apple corer ? (no(n) OR /north/east/south/west/center) : "
read ac
#calculate no of frames to move to frames gw x gh
gwgh=$(($gw * $gh))
echo $gwgh
echo -n "Landscape(l),square(s),portrait(p)? : "
read lsp
#copy originals to $h/originals
cp *.$f $h/originals/
#new stuff should go between here and infinite loop
#infinite loop
lp=0
while [ $lp -lt $lo ]
do
((lp++))
echo "Press Ctrl+C to stop..."
#count how many files we have
cd $h
i=$(ls *.$f | wc -l)
echo $i
#convert everything to specified format or convert then randomise
#randomise yes
echo -n "Randomising "
mogrify -format $t *.$f
find . -maxdepth 1 -name '*.'$t''|while read filename; do echo ${filename}; mv ${filename} ${RANDOM}.$t; done
#crop to 1:1 4:3 or 3:4 depending on lsp before resizing details here https://stackoverflow.com/questions/21262466/imagemagick-how-to-minimally-crop-an-image-to-a-certain-aspect-ratio
if [ $lsp == "s" ] || [ $lsp == "null" ]
then
find . -maxdepth 1 -name '*.'$t''|while read filename; do echo ${filename}; convert ${filename} -gravity center -crop 1:1 swap.$t; mv swap.$t ${filename};done
elif [ $lsp == "l" ]
then
find . -maxdepth 1 -name '*.'$t''|while read filename; do echo ${filename}; convert ${filename} -gravity center -crop 4:3 swap.$t; mv swap.$t ${filename};done
elif [ $lsp == "p" ]
then
find . -maxdepth 1 -name '*.'$t''|while read filename; do echo ${filename}; convert ${filename} -gravity center -crop 3:4 swap.$t; mv swap.$t ${filename};done
fi
#resize for preffered size grid
#mogrify -resize 600x600! -gravity center -extent 600x600 *.$t
#modify to size depending lsp variable
if [ $lsp == "s" ] || [ $lsp == "null" ]
then
mogrify -resize 800x800! *.$t
elif [ $lsp == "l" ]
then
mogrify -resize 800x600! *.$t
elif [ $lsp == "p" ]
then
mogrify -resize 600x800! *.$t
fi
#mogrify -rotate 90 *.$t
#mogrify -flop *.$t
#loop to make montages $gwgh at a time so as not to exhaust imagemagick cache
while [ $i -gt 0 ]
do
#move gwxgh images to frames
find . -maxdepth 1 -name '*.'$t'' | head -n $gwgh | xargs -d $'\n' mv -t $h/frames/
#move to frames directory
cd $h/frames/
d=$(date +%Y-%m-%d_%H%M%S)
montage *.$t -geometry +3+3 -tile $gwx$gh $d.$t
#move output back to source folder
convert $d.$t $d.$f
mv $d.$f $h/rotator
#decrease counter by gwgh
i=$((i-="$gwgh"))
#tidy up
rm *.$t
cd $h
done
cd $h/rotator
if [ $ac == "n" ] || [ $ac == "null" ]
then
mv *.$f $h
elif [ $ac == "center" ]
then
find . -maxdepth 1 -name '*.'$f''|while read filename; do echo ${filename}; convert ${filename} -gravity center -crop 1300x1300+0+0 swap.$f;
mv swap.$f ${RANDOM}${RANDOM}.$f;done
elif [ $ac == "north" ]
then
find . -maxdepth 1 -name '*.'$f''|while read filename; do echo ${filename}; convert ${filename} -gravity north -crop 1300x1300+0+0 swap.$f;
mv swap.$f ${RANDOM}${RANDOM}.$f;done
elif [ $ac == "east" ]
then
find . -maxdepth 1 -name '*.'$f''|while read filename; do echo ${filename}; convert ${filename} -gravity east -crop 1300x1300+0+0 swap.$f;
mv swap.$f ${RANDOM}${RANDOM}.$f;done
elif [ $ac == "south" ]
then
find . -maxdepth 1 -name '*.'$f''|while read filename; do echo ${filename}; convert ${filename} -gravity south -crop 1300x1300+0+0 swap.$f;
mv swap.$f ${RANDOM}${RANDOM}.$f;done
elif [ $ac == "west" ]
then
find . -maxdepth 1 -name '*.'$f''|while read filename; do echo ${filename}; convert ${filename} -gravity west -crop 1300x1300+0+0 swap.$f;
mv swap.$f ${RANDOM}${RANDOM}.$f;done
fi
#mirror vertically
find . -maxdepth 1 -name '*.'$f''|while read filename; do echo ${filename};
convert ${filename} -gravity east -chop 50%x0 output.$f
convert output.$f -flop output2.$f
convert output.$f output2.$f -gravity North +append ${RANDOM}.$f
rm output.$f
rm output2.$f;
done
#mogrify -monochrome *.$f
#uncomment below to create a negative image on each alternate run
#mogrify -negate *.$f
mv *.$f $h
cd $h
# move x no of images to to new folder
new=$(ls *.$f | wc -l)
if (($new > $disin ))
then
dd=$(date +%Y-%m-%d_%H%M%S)
mkdir $h/$dd
find . -maxdepth 1 -name '*.'$f'' | head -n $dis | xargs -d $'\n' mv -t $h/$dd
fi
done
(Below is a modified version for Windows 10 running gitbash with imagemagick installed and on path)
#! /bin/bash
#make directory to hold output
h=$(pwd)
echo $h
#make folder to store originals
mkdir $h/originals
mkdir $h/frames
#make folder to hold output
mkdir rotator
#get formats to use
echo -n "Source image format ? : "
read f
echo -n "Image format to use ? : "
read t
echo -n "Discard cut in ? : "
read disin
echo -n "No to discard ? : "
read dis
#get grid dimensions
echo -n "Grid height ? : "
read gw
echo -n "Grid width ? : "
read gh
echo -n "Number of loops ? : "
read lo
echo -n "Use apple corer ? (no(n) OR /north/east/south/west/center) : "
read ac
#calculate no of frames to move to frames gw x gh
gwgh=$(($gw * $gh))
echo $gwgh
echo -n "Landscape(l),square(s),portrait(p)? : "
read lsp
#copy originals to $h/originals
cp *.$f $h/originals/
#new stuff should go between here and infinite loop
#infinite loop
lp=0
while [ $lp -lt $lo ]
do
((lp++))
echo "Press Ctrl+C to stop..."
#count how many files we have
cd $h
i=$(ls *.$f | wc -l)
echo $i
#convert everything to specified format or convert then randomise
#randomise yes
echo -n "Randomising "
magick mogrify -format $t *.$f
find . -maxdepth 1 -name '*.'$t''|while read filename; do echo ${filename}; mv ${filename} ${RANDOM}.$t; done
#crop to 1:1 4:3 or 3:4 depending on lsp before resizing details here https://stackoverflow.com/questions/21262466/imagemagick-how-to-minimally-crop-an-image-to-a-certain-aspect-ratio
if [ $lsp == "s" ] || [ $lsp == "null" ]
then
find . -maxdepth 1 -name '*.'$t''|while read filename; do echo ${filename}; magick convert ${filename} -gravity center -crop 1:1 swap.$t; mv swap.$t ${filename};done
elif [ $lsp == "l" ]
then
find . -maxdepth 1 -name '*.'$t''|while read filename; do echo ${filename}; magick convert ${filename} -gravity center -crop 4:3 swap.$t; mv swap.$t ${filename};done
elif [ $lsp == "p" ]
then
find . -maxdepth 1 -name '*.'$t''|while read filename; do echo ${filename}; magick convert ${filename} -gravity center -crop 3:4 swap.$t; mv swap.$t ${filename};done
fi
#resize for preffered size grid
#mogrify -resize 600x600! -gravity center -extent 600x600 *.$t
#modify to size depending lsp variable
if [ $lsp == "s" ] || [ $lsp == "null" ]
then
magick mogrify -resize 800x800! *.$t
elif [ $lsp == "l" ]
then
magick mogrify -resize 800x600! *.$t
elif [ $lsp == "p" ]
then
magick mogrify -resize 600x800! *.$t
fi
#magick mogrify -rotate 90 *.$t
#magick mogrify -flop *.$t
#loop to make montages $gwgh at a time so as not to exhaust imagemagick cache
while [ $i -gt 0 ]
do
#move gwxgh images to frames
find . -maxdepth 1 -name '*.'$t'' | head -n $gwgh | xargs -d $'\n' mv -t $h/frames/
#move to frames directory
cd $h/frames/
d=$(date +%Y-%m-%d_%H%M%S)
magick montage *.$t -geometry +3+3 -tile $gwx$gh $d.$t
#move output back to source folder
magick convert $d.$t $d.$f
mv $d.$f $h/rotator
#decrease counter by gwgh
i=$((i-="$gwgh"))
#tidy up
rm *.$t
cd $h
done
cd $h/rotator
if [ $ac == "n" ] || [ $ac == "null" ]
then
mv *.$f $h
elif [ $ac == "center" ]
then
find . -maxdepth 1 -name '*.'$f''|while read filename; do echo ${filename}; magick convert ${filename} -gravity center -crop 1300x1300+0+0 swap.$f;
mv swap.$f ${RANDOM}${RANDOM}.$f;done
elif [ $ac == "north" ]
then
find . -maxdepth 1 -name '*.'$f''|while read filename; do echo ${filename}; magick convert ${filename} -gravity north -crop 1300x1300+0+0 swap.$f;
mv swap.$f ${RANDOM}${RANDOM}.$f;done
elif [ $ac == "east" ]
then
find . -maxdepth 1 -name '*.'$f''|while read filename; do echo ${filename}; magick convert ${filename} -gravity east -crop 1300x1300+0+0 swap.$f;
mv swap.$f ${RANDOM}${RANDOM}.$f;done
elif [ $ac == "south" ]
then
find . -maxdepth 1 -name '*.'$f''|while read filename; do echo ${filename}; magick convert ${filename} -gravity south -crop 1300x1300+0+0 swap.$f;
mv swap.$f ${RANDOM}${RANDOM}.$f;done
elif [ $ac == "west" ]
then
find . -maxdepth 1 -name '*.'$f''|while read filename; do echo ${filename}; magick convert ${filename} -gravity west -crop 1300x1300+0+0 swap.$f;
mv swap.$f ${RANDOM}${RANDOM}.$f;done
fi
#mirror vertically
find . -maxdepth 1 -name '*.'$f''|while read filename; do echo ${filename};
magick convert ${filename} -gravity east -chop 50%x0 output.$f
magick convert output.$f -flop output2.$f
magick convert output.$f output2.$f -gravity North +append ${RANDOM}.$f
rm output.$f
rm output2.$f;
done
#magick mogrify -monochrome *.$f
magick mogrify -negate *.$f
mv *.$f $h
cd $h
# move x no of images to to new folder
new=$(ls *.$f | wc -l)
if (($new > $disin ))
then
dd=$(date +%Y-%m-%d_%H%M%S)
mkdir $h/$dd
find . -maxdepth 1 -name '*.'$f'' | head -n $dis | xargs -d $'\n' mv -t $h/$dd
fi
done